Types - Objects
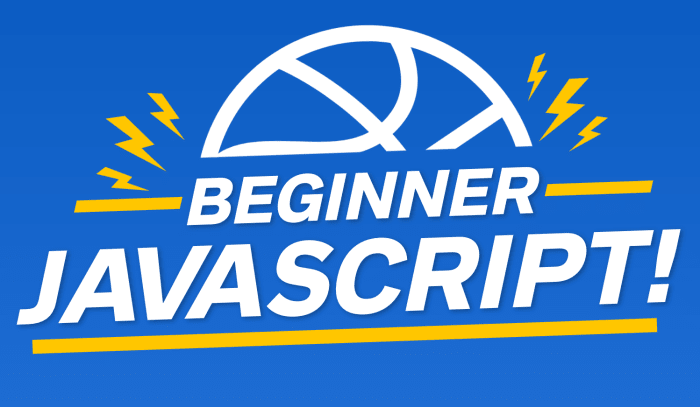
Enjoy these notes? Want to Slam Dunk JavaScript?
These are notes based on my Beginner JavaScript Video Course. It's a fun, exercise heavy approach to learning Modern JavaScript from scratch.
Use the code BEGINNERJS for an extra $10 off.
Objects in JavaScript are the biggest building block and almost everything in JavaScript is an object.
Objects are used for collections of data or collections of functionality.
We will be going through objects in depth in this course, there is an entire section dedicated to them and we will be using objects throughout all of our examples.
For now, you need to know that when something is an object in JavaScript, it's because we want to group things together.
Up to now, we have been using random variables like const name = 'wes';
or const age = 100;
. That is not the best way to do things, because the values are not associated.
What we can do instead is create an object called person.
We will create it using two curly brackets and a semi colon. That is the most common way to make an object, but there are other ways that we will go over.
const person = {};
Inside of the person object, you have what are called properties and values. Add the following to types.js
👇
const person = {
first: "wes",
last: "bos",
age: 100,
};
What we have done here is created an object that allows us to group together variables. In the example above we have first, last and age variables all contained within an object for a collection that is a "person".
In the console, if you type person it will return the value, which is the object. If you check the type of the person variable, it will return the object type.
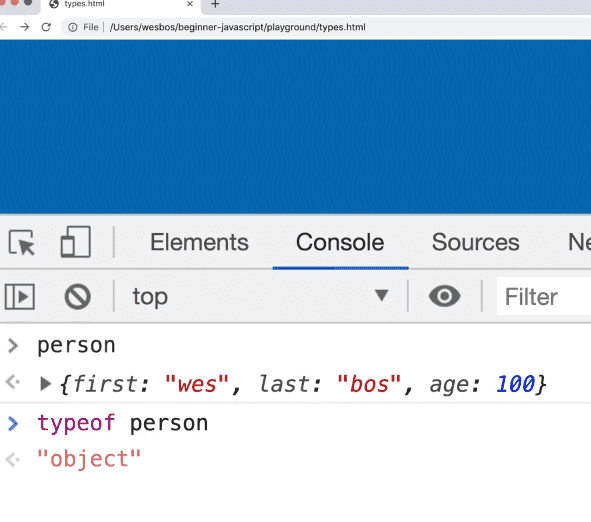
You can expand the object in the console to see it like so 👇
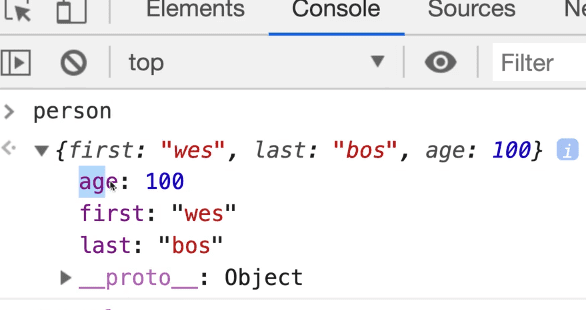
You may notice that the object properties are in a different order then we put them in within types.js
. We will go over that after. The short and skinny is that the order doesn't matter in an object. If you need the order to matter, use an array or Map data structure.
To access the properties, there is a couple of different ways we can do it.
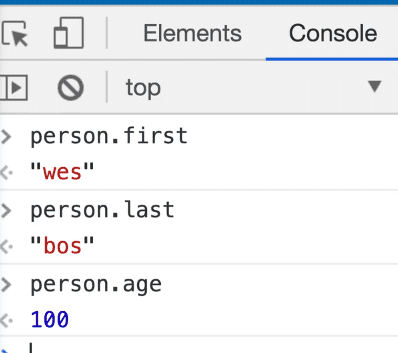
We are using the dot notation
in the examples above.
When we get deeper into objects in the future, we will go over the other ways to do it as well as things like nesting objects and object vs reference and copying objects.
The last type we need to talk is Symbols.
What you need to know about it right now is that it is a way to do unique properties (or unique identifiers in general in JavaScript).
There is more to it, but it's complex and typically used by more advanced users (Wes barely ever uses them) so that is all we need to know for now.
Find an issue with this post? Think you could clarify, update or add something?
All my posts are available to edit on Github. Any fix, little or small, is appreciated!